Creating a Kirby-powered blog
In this section you’ll learn how to build a blog with Kirby. We’ll start with a very basic and minimalistic blog here. You will find more advanced stuff in related sections.
Setting up the content
For this example we will use a very simple file structure setup. You can definitely get way more fancy than that, but let’s keep it simple:
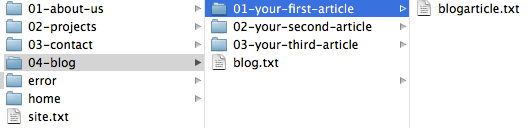
First we add a new visible blog folder to our site (04-blog) so it will be visible in our menu. Inside that blog folder we add subfolders for each new article and a blog.txt
.
blog.txt
Add two fields to your blog.txt
:
Title: Blog
----
Text: This is a nice Demo Blog.
----
More on how we use that later when we build our templates.
Articles
Inside each article folder, add a blogarticle.txt
. By naming it like this, we can later add a blogarticle.php
template to our template folder and create a specific template for all articles.
Add three fields to your blogarticle.txt
Title: This is my first Article
----
Date: 08.12.2011
----
Text: Hello World!
----
Building the templates
Add a blog.php
and a blogarticle.php
to site/templates
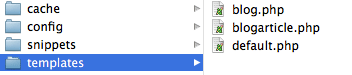
blog.php
This is the template for http://yourdomain.com/blog
, which will show
a list of all articles.
<?php snippet('header') ?>
<?php snippet('menu') ?>
<section class="content blog">
<h1><?= $page->title()->html() ?></h1>
<?= $page->text()->kirbytext() ?>
<?php foreach($page->children()->visible()->flip() as $article): ?>
<article>
<h1><?= $article->title()->html() ?></h1>
<p><?= $article->text()->excerpt(300) ?></p>
<a href="<?= $article->url() ?>">Read more…</a>
</article>
<?php endforeach ?>
</section>
<?php snippet('footer') ?>
Breaking it down a bit…
First we include our header and main menu snippets and start the content section.
By giving the content section a second blog
selector we can easily style it later in
our css.
<?php snippet('header') ?>
<?php snippet('menu') ?>
<section class="content blog">
Next we build the title of our blog and the short description, which we’ve added in blog.txt
<h1><?= $page->title()->html() ?></h1>
<?= $page->text()->kirbytext() ?>
The next few lines of code is a loop, where we build a short version for each article of our blog:
<?php foreach($page->children()->visible()->flip() as $article): ?>
<article>
<h1><?= $article->title()->html() ?></h1>
<p><?= $article->text()->excerpt(300) ?></p>
<a href="<?= $article->url() ?>">Read more…</a>
</article>
<?php endforeach ?>
Breaking it down some more…
With $page->children()->visible()
we get a set of all visible subpages in our blog folder. This will return the subfolders we got so far in the following order:
01-your-first-article
02-your-second-article
03-your-third-article
But for our blog we want the latest post to be on top, which is 03-your-third-article.
So what we do is to add ->flip()
: $page->children()->visible()->flip
, which will return the set of pages in reverse order.
03-your-third-article
02-your-second-article
01-your-first-article
Now we can build our foreach loop with that:
<?php foreach($page->children()->visible()->flip() as $article): ?>
…
<?php endforeach ?>
Within the foreach loop, we build the html for each article in our list.
<?php foreach($page->children()->visible()->flip() as $article): ?>
<article>
<h1><?= $article->title()->html() ?></h1>
<p><?= $article->text()->excerpt(300) ?></p>
<a href="<?= $article->url() ?>">Read more…</a>
</article>
<?php endforeach ?>
Let’s have a close look at the following line:
<p><?= $article->text()->excerpt(300) ?></p>
In our article list, we don’t want to show the entire content of each article. That would totally mess up our overview. Kirby has a nice little excerpt()
function, which makes it easy to only show the first few words or sentences of a large article, which is just perfect for our article overview list.
With $article->text()->excerpt(300)
we get an excerpt with a maximum of 300 characters for each article.
With the next line…
<a href="<?= $article->url() ?>">Read more…</a>
…we finally link to the full article. For example:
http://yourdomain.com/blog/your-first-article
This is where we need the blogarticle.php
template, which we will build next…
blogarticle.php
This is going to be super easy. Open blogarticle.php
in your editor. It will be responsible for displaying the detailed view of articles.
The template code for this is very short and easy to understand.
<?php snippet('header') ?>
<?php snippet('menu') ?>
<section class="content blogarticle">
<article>
<h1><?= $page->title()->html() ?></h1>
<?= $page->text()->kirbytext() ?>
<a href="<?= url('blog') ?>">Back…</a>
</article>
</section>
<?php snippet('footer') ?>
All you need to do is to include the header and menu again as well as the footer snippet at the bottom. In between just add a content section and give it a blogarticle selector so we can add some nice css again later.
The rest is super straight forward. Add the title of your article and the full text of your article and you’re done.
You might also want to add a back link:
<a href="<?= url('blog') ?>">Back…</a>
which will take your visitors back to the article list.
Add an intro to your articles
To add an article intro which you can display and maybe style differently from the rest of the post you have to set up an additional field in your .blogarticle.txt
first.
Title: Some title
----
Text: Some great text
----
Tags: design, photography, architecture, whatever
----
Intro: This is a nice article intro. Lorem ipsum dolor sit amet, consetetur sadipscing elitr, sed diam nonumy eirmod tempor invidunt ut labore et dolore magna aliquyam erat, sed diam voluptua.
----
Text: […]
Template adjustments
Now we are going to implement the intro in the blog’s overview loop (instead of an excerpt) like this:
<?php snippet('header') ?>
<?php snippet('menu') ?>
<main class="blog" role="main">
<h1><?= $page->title()->html() ?></h1>
<?= $page->text()->kirbytext() ?>
<?php foreach($page->children()->visible()->flip() as $article): ?>
<article>
<h1><?= $article->title()->html() ?></h1>
<?= $article->intro()->kirbytext() ?>
<a href="<?= $article->url() ?>">Read more…</a>
</article>
<?php endforeach ?>
</main>
<?php snippet('footer') ?>
Your article template would look like this:
<?php snippet('header') ?>
<?php snippet('menu') ?>
<main class="blogarticle" role="main">
<article>
<h1><?= $page->title()->html() ?></h1>
<?= $page->intro()->kirbytext() ?>
<?= $page->text()->kirbytext() ?>
<a href="<?= url('blog') ?>">Back…</a>
</article>
</main>
<?php snippet('footer') ?>